onclick
Archived blogs from here
The onclick property is an event handler for processing click events on the specific element.
The syntax looks this
element.onclick = makeChange;
function makeChange() {
// do something
}
or;
element.onclick = function makeChange() {
// do something
};
addEventListener
The addEventListener() method of the EventTarget interface sets up a function that will be called whenever the specified event is delivered to the target. β MDN
The syntax looks like this
addEventListener(type, listener);
addEventListener(type, listener, options);
addEventListener(type, listener, useCapture);
As you can see, the addEventListener
takes 3 arguments
- type: this is the event type, such as
click
,keydown
,mouseover
and so on. - listener: it is usually the function that you had declared, such as change background colour, text colour, and many options
function makeChange() {
// do something
}
element.addEventListener("click", makeChange);
-
options:
- capture: defaults to
false
, if true, it will capture all nested elements from the top of the DOM tree to the clicked element - once: defaults to
false
, if true, the element only can be clicked once. - passive: defaults to
false
, if true, the specific function will never call preventDefault() - signal: the listener will be removed when the signal is given.
- capture: defaults to
-
useCapture: this is similar to the capture from options category.
What are the differences between capture
and useCapture
<body>
<div class="one">
<div class="two">
<div class="three"></div>
</div>
</div>
</body>
function logText() {
console.log(this.classList.value);
}
// capture
divs.forEach((div) => {
div.addEventListener("click", logText, { capture: true });
});
// useCapture
divs.forEach((div) => {
div.addEventListener("click", logText, { useCapture: true });
});
Result table - the order of logging after clicked
value | capture | useCapture |
---|---|---|
true | one -> two -> three | three -> two -> one |
false | three -> two -> one | three -> two -> one |
%[https://codepen.io/victoriacheng15/pen/eYejyjr]
Differences between addEventListener and onclick
type | addEventListener | onclick |
---|---|---|
number of event | many events | single event |
event propagation | can control with 3rd argument | cant control |
compatibility | Doesnβt work on older IE | works on all browsers |
browser support
You can check this Can I Use site to see what browser version support certain property.
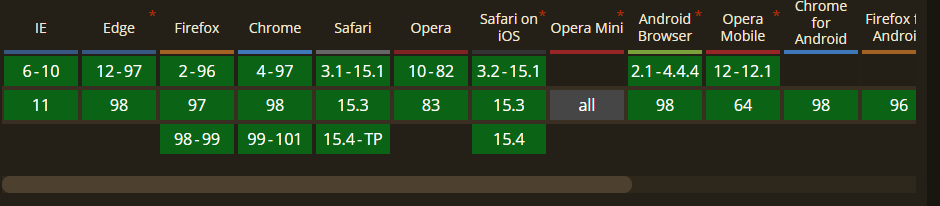
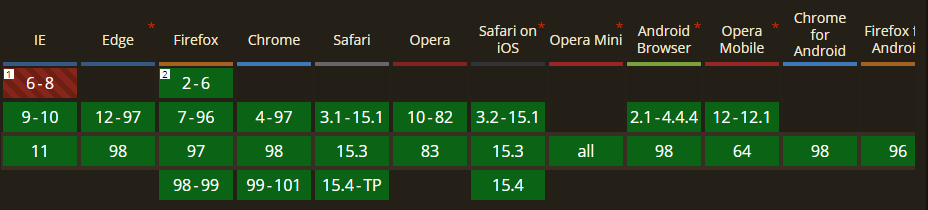
sources:
- MDN - onclick
- MDN - addEventListener
- JavaScript Info - bubbling and capturing
- Difference between addEventListener and onclick in JavaScript
Recap
Both onclick and addEventlistener are supported on almost all browsers beside Internet Explorer version 6 - 8
. But, which one to use? It boils down to what are you trying to achieve. Does it do one thing only, you may go with onclick for a short and simple way to write. However, addEventListener is recommended to use.
Thank you!
Thank you for reading my blog! π